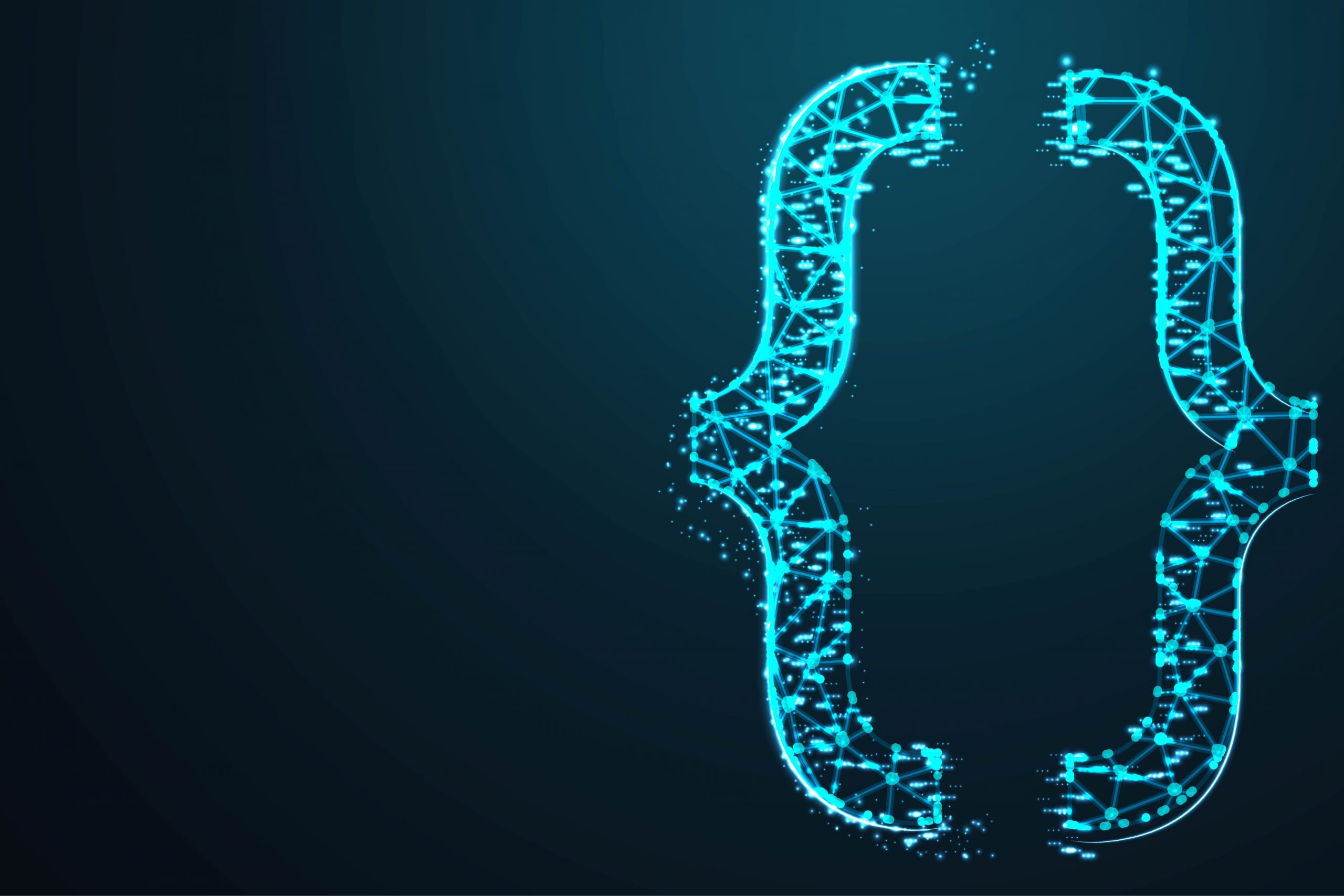
Python: 7 Tips And Tricks To Write Better Code
Learn how to code in Python way...
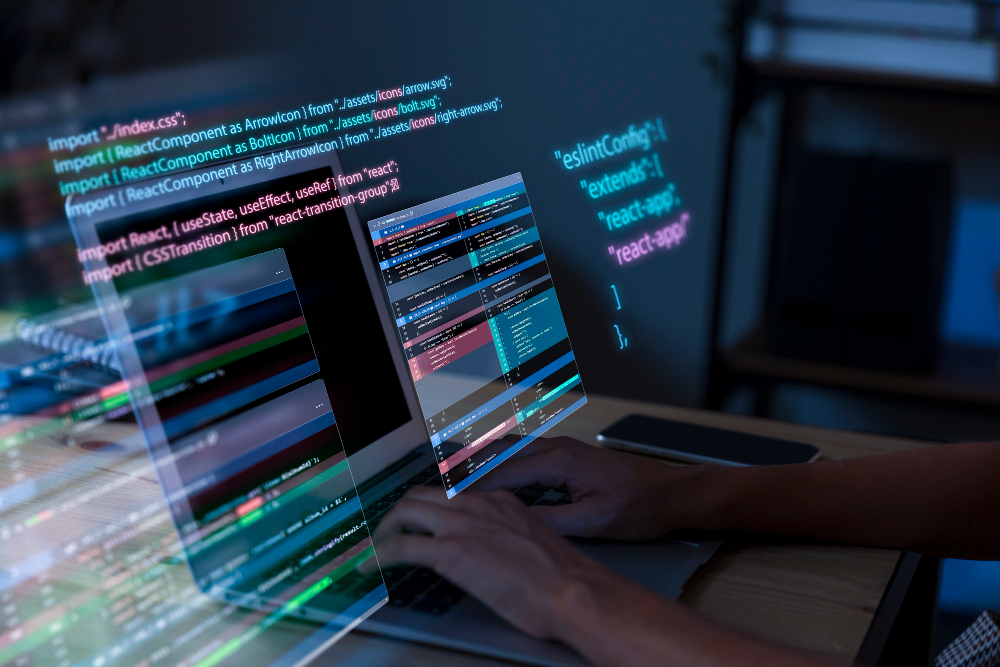
Coding is not 'fun', it's technically and ethically complex. It demands intense focus. Python programming is no different. You can make it fun with the help of some tips and tricks.
Python is a flexible language that provides many different ways to accomplish the same functionalities. With better organization, you can write clear code in Python. The best practice while writing better Python code is to make your code readable.
Tips and Tricks to Write the Better Python Code
- Negative Indexing: Python supports negative indexing, so we can use -1 to refer to the last element in the sequence and count the items backward.
... data_shape = (100, 50, 4) ... names = ["John", "Aaron", "Mike", "Danny"] ... print(names[-3:-1]) o/p ['Aaron', 'Mike']
2. Use Built-in Functions: There are 68 built-in python functions. They save our time but also help us avoid unnecessary lines of code and make your code elementary and more proficient.
Eg: max()
, min()
, sum()
, len()
3. Use Default Arguments: Default arguments make your code more concise.
def wish(name='World'):
print(f'Hello {name}!')
wish()
4. Use Docstrings: These string literals that appear right after the definition of a function, method, class, or module.
def
my_function():
'''Demonstrates triple double quotes
docstrings and does nothing really.'''
return
None
print
(
"Using __doc__:"
)
print
(my_function.__doc__)
print
(
"Using help:"
)
help(my_function)
5. Use Meaningful Variable Names: Use variable names with context. Avoid using acronyms or abbreviations.
Eg: “the first value” and “the second value,” instead of "x1" and "x2"
6. Securing User Input With GetPass: The getpass module provides a portable way to handle such password prompts securely.
import
getpass
password
=
getpass.getpass(
"Enter your password: "
)
print("Your password is:", password)
7. Don’t Repeat Yourself (DRY): It is the best practice in software development that recommends software engineers to do something once, and only once. Keep your code clean, readable, and easy to understand.
Conclusion
Python popularity still soaring and will continue in the future. For future and present Python programmers, these tips and tricks will help you to write better Python code with fewer chances of bugs occurring.